vue中el-checkbox全选、反选、多选的实现
脚本之家 / 编程助手:解决程序员“几乎”所有问题!
脚本之家官方知识库 → 点击立即使用
vue el-checkbox全选、反选、多选
描述:实现对一组数据进行全选,反选、多选操作
- 全选
1 2 3 4 5 | handleCheckAllChange(val) { this .checkedCities = val ? cityOptions : []; this .isIndeterminate = false ; this .checkInvert = false ; } |
- 反选
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | handleInvertCheckChange(val) { let cities = this .cities; let checkedCities = this .checkedCities; if (checkedCities.length === 0) { checkedCities = val ? cities : []; } else if (checkedCities.length === cities.length) { this .checkedCities = []; this .checkAll = false ; } else { let list = cities.concat(checkedCities).filter((v, i, array) => { return array.indexOf(v) === array.lastIndexOf(v); }); this .checkedCities = list; } } |
- 多选
1 2 3 4 5 6 7 | handleCheckedCitiesChange(value) { let checkedCount = value.length; this .checkAll = checkedCount === this .cities.length; this .isIndeterminate = checkedCount > 0 && checkedCount < this .cities.length; this .checkInvert = false ; } |
完整代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | <template> <div> <el-checkbox v-model= "checkAll" @change= "handleCheckAllChange" :indeterminate= "isIndeterminate" >全选</el-checkbox > <el-checkbox v-model= "checkInvert" @change= "handleInvertCheckChange" >反选</el-checkbox > <el-checkbox-group v-model= "checkedCities" @change= "handleCheckedCitiesChange" > <el-checkbox v- for = "city in cities" :label= "city" :key= "city" >{{ city }}</el-checkbox> </el-checkbox-group> </div> </template> <script> const cityOptions = [ "上海" , "北京" , "广州" , "深圳" ]; export default { data() { return { checkAll: false , checkInvert: false , checkedCities: [ "上海" , "北京" ], cities: cityOptions, isIndeterminate: true , }; }, methods: { // 全选 handleCheckAllChange(val) { this .checkedCities = val ? cityOptions : []; this .isIndeterminate = false ; this .checkInvert = false ; }, // 反选 handleInvertCheckChange(val) { let cities = this .cities; let checkedCities = this .checkedCities; if (checkedCities.length === 0) { checkedCities = val ? cities : []; } else if (checkedCities.length === cities.length) { this .checkedCities = []; this .checkAll = false ; } else { let list = cities.concat(checkedCities).filter((v, i, array) => { return array.indexOf(v) === array.lastIndexOf(v); }); this .checkedCities = list; } }, // 多选 handleCheckedCitiesChange(value) { let checkedCount = value.length; this .checkAll = checkedCount === this .cities.length; this .isIndeterminate = checkedCount > 0 && checkedCount < this .cities.length; this .checkInvert = false ; }, }, }; </script> <style> </style> |
checkbox多选框,indeterminate 状态
举个例子。比如选择星期。一周七天
2种方法。思密达。。。。第一种带局限性。笨办法,也发出来大家看看(推荐使用第二种)
这是方式的值是组件自带的值方式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | <template> <div> <el-checkbox :indeterminate= "isIndeterminate" v-model= "checkAll" @change= "handleCheckAllChange" >全选</el-checkbox> <div style= "margin: 15px 0;" ></div> <el-checkbox-group v-model= "checkedCities" @change= "handleCheckedCitiesChange" > <el-checkbox v- for = "city in cities" :label= "city" :key= "city" >{{city}}</el-checkbox> </el-checkbox-group> </div> </template> <script> const cityOptions = [ '周一' , '周二' , '周三' , '周四' , '周五' , '周六' , '周天' ] export default { data() { return { checkAll: false , checkedCities: [], cities: cityOptions, isIndeterminate: true , arr:[] } }, methods: { handleCheckAllChange(val) { let tempArr = [] this .checkedCities = val ? cityOptions : [] this .isIndeterminate = false // console.log(this.checkedCities) // console.log(val) if ( this .checkedCities != []) { if (val == true ){ tempArr = [1,2,3,4,5,6,0] this .arr = tempArr } else if (val== false ){ tempArr = [] this .arr = tempArr } } console.log( this .arr) }, handleCheckedCitiesChange(value) { let checkedCount = value.length; this .checkAll = checkedCount === this .cities.length; this .isIndeterminate = checkedCount > 0 && checkedCount < this .cities.length; var tempArr = [] for (let i=0;i<value.length;i++){ if (value[i] === '周一' ) { tempArr.push(1) } else if (value[i] === '周二' ){ tempArr.push(2) } else if (value[i] === '周三' ){ tempArr.push(3) } else if (value[i] === '周四' ){ tempArr.push(4) } else if (value[i] === '周五' ){ tempArr.push(5) } else if (value[i] === '周六' ){ tempArr.push(6) } else if (value[i] === '周天' ){ tempArr.push(0) } } this .arr = tempArr console.log( this .arr) } } } </script> <style scoped> </style> |
之后UP想了一下。不对。后台反过来的数组不应该是这种。大部分都应该是obj的形式
于是乎。
1 | const cityOptions = [{a: '周一' ,b:1}, {a: '周二' ,b:2}, {a: '周三' ,b:3},{a: '周四' ,b:4},{a: '周五' ,b:5},{a: '周六' ,b:6},{a: '周天' ,b:7}]; |
对吧这种形式才对。说不定可能有很多很多。万一叫你把一个月都列出来也说不定。
继续上代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 | <template> <div style= "height: 1000px" > <el-checkbox :indeterminate= "isIndeterminate" v-model= "checkAll" @change= "handleCheckAllChange" >全选</el-checkbox> <div style= "margin: 15px 0;" ></div> <el-checkbox-group v-model= "checkedCities" @change= "handleCheckedCitiesChange" > <el-checkbox v- for = "city in cities" :label= "city.b" :key= "city.b" >{{city.a}}</el-checkbox> </el-checkbox-group> </div> </template> <script> const cityOptions = [{a: '周一' ,b:1}, {a: '周二' ,b:2}, {a: '周三' ,b:3},{a: '周四' ,b:4},{a: '周五' ,b:5},{a: '周六' ,b:6},{a: '周天' ,b:7}]; export default { name: "tourSpecialEdition" , components: {}, data(){ return { checkAll: false , checkedCities: [], cities: cityOptions, isIndeterminate: false } }, created() {}, mounted() {}, methods: { handleCheckAllChange(val) { const arr = val ? cityOptions : []; this .checkedCities = []; arr.map(item => { this .checkedCities.push(item.b); console.log( this .checkedCities.sort()) }); this .isIndeterminate = false ; }, handleCheckedCitiesChange(value) { let arrTime = value this .checkedCities = arrTime console.log( this .checkedCities.sort()) let checkedCount = value.length; this .checkAll = checkedCount === this .cities.length; this .isIndeterminate = checkedCount > 0 && checkedCount < this .cities.length; } } } </script> <style scoped> </style> |
总结
以上为个人经验,希望能给大家一个参考,也希望大家多多支持脚本之家。
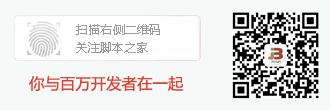
微信公众号搜索 “ 脚本之家 ” ,选择关注
程序猿的那些事、送书等活动等着你
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。
如若内容造成侵权/违法违规/事实不符,请将相关资料发送至 reterry123@163.com 进行投诉反馈,一经查实,立即处理!
最新评论